Inline in C++ vs. Macro in C++ — What's the Difference?

By Tayyaba Rehman — Published on January 11, 2024
Inline in C++ is a function qualifier for efficiency, integrating code at compile-time. Macro in C++ is a preprocessor directive for text substitution, done before compilation.
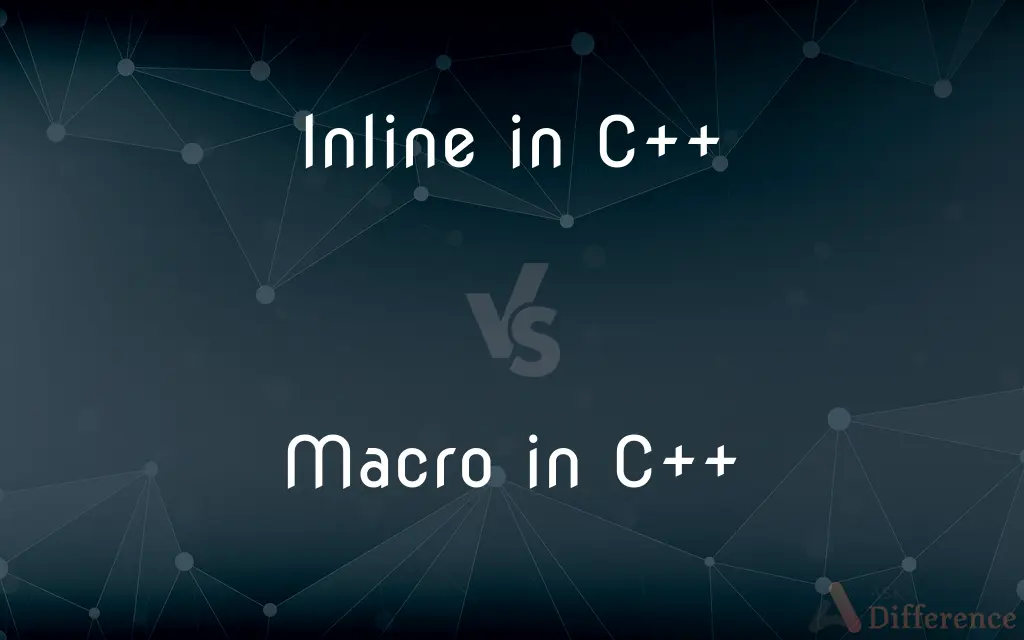
Difference Between Inline in C++ and Macro in C++
Table of Contents
ADVERTISEMENT
Key Differences
Inline in C++ is a function specifier that advises the compiler to insert the function's code at each call site, potentially reducing the overhead of function calls. In contrast, Macro in C++ is a preprocessor feature that replaces macro names with specified code or values before the program is compiled, working as a text substitution tool.
Inline functions retain type safety and debugging capabilities, as they are part of the C++ language and follow its rules. However, Macros lack type safety and can lead to errors if not used carefully, as they are simple text replacements without understanding the context or syntax of the code.
Inline functions are evaluated by the compiler, which may ignore the inline request if the function is too complex or large. On the other hand, Macros are always expanded by the preprocessor, regardless of their size or complexity, leading to potential code bloat.
Inline functions allow for more readable and maintainable code since they follow the normal function syntax and semantics. In contrast, Macros can make the code more difficult to read and debug, especially when they are complex or extensively used.
Inline functions are more modern and preferred for optimizing function calls in C++, while Macros are often used for conditional compilation, constants, and simple repetitive tasks. Macros have a wider range of use cases but come with risks associated with their untyped nature.
ADVERTISEMENT
Comparison Chart
Nature
Function specifier, part of C++ language
Preprocessor directive, text substitution tool
Type Safety
Maintains type safety
Lacks type safety
Compiler vs. Preprocessor
Evaluated and managed by the compiler
Handled by the preprocessor
Readability
Generally more readable and maintainable
Can be less readable and harder to debug
Common Use
Optimizing function calls
Conditional compilation, constants, repetitions
Compare with Definitions
Inline in C++
Inline functions follow normal function syntax and rules.
Inline functions are easier to debug than macros.
Macro in C++
Macros perform text substitution before compilation.
#define PI 3.14
Inline in C++
Inline functions maintain type safety.
Inline functions prevent type-related errors.
Macro in C++
Useful for conditional compilation and simple tasks.
#ifdef DEBUG for debugging purposes.
Inline in C++
Inline functions suggest code integration at the call site.
Inline int square(int x) { return x * x; }
Macro in C++
Macros can make the code harder to read and debug.
Complex macros can obscure code understanding.
Inline in C++
They potentially reduce the overhead of function calls.
Inline functions can make small functions faster.
Macro in C++
They lack type safety and context understanding.
Macros can lead to unexpected errors if misused.
Inline in C++
The compiler may ignore the inline request for complex functions.
Complex inline functions might not be inlined.
Macro in C++
Macros are always expanded by the preprocessor.
Macros can result in code bloat.
Common Curiosities
Can the compiler ignore an inline request?
Yes, the compiler may choose not to inline complex or large functions.
When should I use inline functions?
Use inline for small, frequently called functions to reduce call overhead.
Why are macros used in C++?
Macros are used for conditional compilation, constants, and simple repetitive code.
Can inline functions lead to code bloat?
Overusing inline functions, especially with large functions, can lead to code bloat.
What is an inline function in C++?
An inline function advises the compiler to insert the function's code at the call site.
What is a macro in C++?
A macro is a preprocessor directive for text substitution in code.
Can I pass function pointers to inline functions?
Yes, but the function may not be inlined if passed as a pointer.
Are macros type-safe in C++?
No, macros lack type safety and can lead to errors.
Do inline functions improve performance?
They can improve performance by eliminating the overhead of function calls.
Are macros expanded during compile time?
Macros are expanded by the preprocessor before the compilation process.
Is debugging easier with inline functions or macros?
Debugging is generally easier with inline functions due to better integration with C++.
Can inline functions be recursive?
Yes, but the compiler may not inline recursive calls.
Do inline functions maintain scope rules?
Yes, inline functions follow the same scope rules as regular functions.
Are macros part of the C++ language?
Macros are part of the preprocessor, not the C++ language itself.
How do macros affect code readability?
Complex macros can make code less readable and harder to debug.
Share Your Discovery
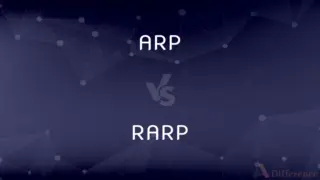
Previous Comparison
ARP vs. RARP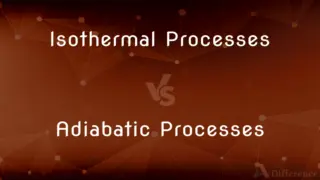
Next Comparison
Isothermal Processes vs. Adiabatic ProcessesAuthor Spotlight

Written by
Tayyaba RehmanTayyaba Rehman is a distinguished writer, currently serving as a primary contributor to askdifference.com. As a researcher in semantics and etymology, Tayyaba's passion for the complexity of languages and their distinctions has found a perfect home on the platform. Tayyaba delves into the intricacies of language, distinguishing between commonly confused words and phrases, thereby providing clarity for readers worldwide.