& vs. && — What's the Difference?

By Tayyaba Rehman — Published on January 13, 2024
'&' is a bitwise AND operator; '&&' is a logical AND operator, often controlling flow with short-circuiting.
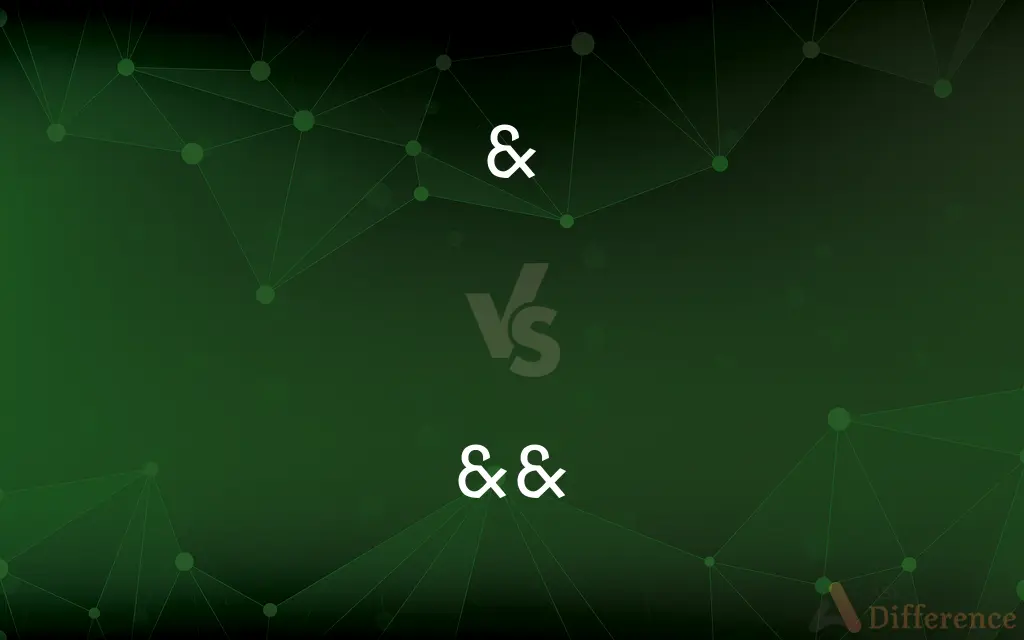
Difference Between & and &&
Table of Contents
ADVERTISEMENT
Key Differences
The '&' operator is used in programming languages to perform a bitwise AND operation, where each bit of the result is 1 if the corresponding bits of both operands are 1. The '&&' operator, however, is a logical AND that evaluates to true if both operands are true and false otherwise.
In the context of control flow, using '&' will result in the evaluation of both sides of the expression, which can be unnecessary or inefficient if the first operand is false. '&&', on the other hand, will short-circuit, meaning if the first operand evaluates to false, the second operand is not evaluated.
The '&' operator can also function as a logical operator in some languages, but it does not short-circuit. In contrast, '&&' is strictly a logical operator and always employs short-circuit evaluation in logical expressions.
When programming in C or similar languages, '&' is often used in bit manipulation tasks, such as setting or clearing specific bits within a byte. '&&' is typically used in conditional statements, like those found in if-else structures, to combine multiple boolean expressions.
In multi-threading contexts, especially in Java, '&' can be used with boolean values in condition checks within synchronized blocks where short-circuiting is not desired, ensuring all conditions are checked. '&&' is commonly used in conditions that, once false, render subsequent checks irrelevant.
ADVERTISEMENT
Comparison Chart
Operation Type
Bitwise operation on binary representations.
Logical operation on boolean values.
Short-Circuit Behavior
Does not short-circuit; evaluates both operands.
Short-circuits; evaluates second operand if needed.
Usage in Conditional Statements
Rarely used; does not short-circuit.
Commonly used for its short-circuiting.
Result
Returns a numerical value after the bitwise AND.
Returns a boolean value.
Use in Multi-threading
Can be used when all conditions must be evaluated.
Used when evaluation can stop at the first false.
Compare with Definitions
&
Can serve as a logical AND in absence of short-circuiting.
If (check1() & check2()) { //... } ensures both methods are called.
&&
Used in conditions that only execute if all operands are true.
If (isValid && isWithinRange) { //... } checks both conditions.
&
In expressions, evaluates both sides regardless of the first operand's truth.
True & false will evaluate both expressions.
&&
A logical operator that performs short-circuit AND operation.
If (condition1 && condition2) { //... } may skip condition2.
&
Used for setting, clearing, and toggling bits.
Flags = flags & ~mask; clears the bits specified by mask.
&&
In programming, controls flow by combining boolean expressions.
While (keepRunning && hasData) { //... } continues if both are true.
&
A bitwise operator that performs AND on each bit pair.
0xF4 & 0xFF results in 0xF4.
&&
Evaluates to true if both boolean expressions are true.
True && true evaluates to true.
&
In C/C++, can reference an address of a variable.
Int a = 42; int *ptr = &a; assigns the address of a to ptr.
&&
Commonly used in if-else structures for multiple conditions.
If (userInput && userInput.isValid()) { //... } checks for non-null and validity.
Common Curiosities
Can & be used for boolean AND operations?
Yes, but it doesn't short-circuit and is not common for boolean logic.
What is short-circuiting?
Short-circuiting is when logical evaluation stops if the outcome is determined early.
What does the & operator do?
The & operator performs a bitwise AND on two numeric operands.
When do I use && in my code?
Use && to combine boolean expressions in conditional statements.
Can && be used to optimize performance?
Yes, by avoiding unnecessary evaluations when the result is already known.
Is && a bitwise operator?
No, && is strictly a logical operator.
In what scenario might & be preferred over &&?
When every condition must be checked, such as in some hardware-level operations.
Can && be used with non-boolean values?
In languages like C, non-zero values are treated as true, and zero as false.
Are & and && interchangeable?
No, they serve different purposes and are not directly interchangeable.
Does & evaluate both operands even if the first is false?
Yes, & evaluates both operands regardless of the first one's value.
Is & used in high-level programming?
It's less common than && but is used for bit manipulation.
Does & work with floating-point numbers?
No, it's not intended for use with floating-point numbers.
Does & have any special meaning in C pointers?
Yes, it's used to get the address of a variable.
Is && more efficient than & in logical operations?
Yes, because it doesn't evaluate the second operand if the first is false.
Does && work in all programming languages?
Most C-like languages support &&, but its implementation may vary in others.
Share Your Discovery

Previous Comparison
May I vs. Can I
Next Comparison
2 Star Hotels vs. 3 Star HotelsAuthor Spotlight

Written by
Tayyaba RehmanTayyaba Rehman is a distinguished writer, currently serving as a primary contributor to askdifference.com. As a researcher in semantics and etymology, Tayyaba's passion for the complexity of languages and their distinctions has found a perfect home on the platform. Tayyaba delves into the intricacies of language, distinguishing between commonly confused words and phrases, thereby providing clarity for readers worldwide.